Monday, February 16, 2009
Wonderful slow-motion stabilized video montage of New York
New York 2008 from Vicente Sahuc on Vimeo.
Bermain dengan Timer 1 AVR

Yang namanya timer sering kali kita gunakan. Misal saat mau nampilin rpm, kita butuh timer sebagai acuan. Atau untuk menghidupkan device dengan interval tertentu.
AVR yang saya pakai sebagai contoh adalah ATmega 8535. AVR ini memilki 3 timer. Yaitu:
- TIMER 0 (8 bit)
- TIMER 1 (16 bit)
- TIMER 2 (8 bit)
Apa yang dimaksud timer 8 bit dan 16 bit?
timer 8 bit adalah timer yg bisa mencacah/menghitung sampai maksimal nilai 0xFF heksa (dalam biner = 1111 1111). Pada ATmega 8535 ada 2 timer jenis ini yaitu TIMER 0 dan 2
Klo yg 16 bit nilai maksimalnya 0xFFFF. Pada ATmega8535 timer jenis 16 bit adalah TIMER 1. Artikel kali ini akan membahas TIMER 1.
Dulu ak disaranin klo timer mau presisi harus memakai bahasa assembly. Hitung jumlah instruksi yg kita tulis. lalu hitung lama waktunya. Hmmmm.. ribet bener...
Untung aja nemu artikel tentang interrupt timer. Dengan Interrupt kita gak perlu susah2 menghitung berapa waktu yang di perlukan untuk meng eksekusi seluruh program kita. Karena saat program dijalanin, timer juga jalan sendiri (digerakkan XTAL). Trus saat nilai tercapai terjadilah interrupt timer.
Register yg biasa saya gunakan untuk menset nilai Timer1 adalah register TCNT, register TCNT sendiri dibagi dua: TCNT 1 H dan TCNT 1 L.
rumus yang digunakan adalah :
TCNT = (1+0xFFFF) - (waktu *( XTAL / prescaler) )
waktu --> waktu yg kita inginkan
XTAL --> frekuensi xtal yg dipakai
prescaler --> nilai prescaler
Apa nilai prescaler itu?
Timer membutuhkan clock source. Biasanya clock source yg saya pakai adalah clock sistem (XTAL). Dan kita bisa menset besarnya nilai ini. Maximum sama dengan XTAL, minimum XTAL/1024. Nah nilai pembagi (1024) ini yg disebur nilai prescaler.
Macam2 nilai prescaler yg diijinkan: 1, 8 , 64 , 256 , 1024
Untuk mengubah nilai prescaler timer 1, kita harus merubah nilai register TCCR1B bit 0...2
gambar diatas di ambil dari data sheet ATmega 8535 hal.113
Contoh Program:
Mengakses Timer 1 dengan interval waktu 1 detik.
#include <mega8535.h>
#include <stdio.h>// LCD di PORT B
#asm
.equ __lcd_port=0x18
#endasm
#include <lcd.h>
unsigned char buff[30];
unsigned long detik;
// sub rutin saat terjadi interrupt Timer 1
interrupt [TIM1_OVF] void timer1_ovf_isr(void)
{
TCNT1H=0xC2;
TCNT1L=0xF7;
detik++;
lcd_clear();
sprintf(buff,"detik %d",detik);
lcd_puts(buff);
}void main(void)
{// Timer/Counter 1 initialization
// Clock source: System Clock
// Clock value: kHz
// Mode: Normal top=FFFFh
// OC1A output: Discon.
// OC1B output: Discon.
// Noise Canceler: Off
// Input Capture on Falling Edge
TCCR1A=0x00;
TCCR1B=0x04;
TCNT1H=0xC2;
TCNT1L=0xF7;
OCR1AH=0x00;
OCR1AL=0x00;
OCR1BH=0x00;
OCR1BL=0x00;// Timer(s)/Counter(s) Interrupt(s) initialization
TIMSK=0x04;// Analog Comparator initialization
// Analog Comparator: Off
// Analog Comparator Input Capture by Timer/Counter 1: Off
// Analog Comparator Output: Off
ACSR=0x80;
SFIOR=0x00;// LCD module initialization
lcd_init(16);// Global enable interrupts
#asm("sei")
lcd_putsf("wait...");
while (1)
{
};
}
Program di atas menggunakan timer 1 untuk menambah nilai variabel "detik" setiap 1 detik sekali. Kemudian menampilkan hasilnya ke LCD.
ayo kita mutilasi code program di atas:
yang akan kita bahas dari program diatas adalah code yang kliatan ruwet aja. Klo yg biasa silahkan lihat di artikel2 sebelumnya .. ^_^
**************
// LCD di PORT B
#asm
.equ __lcd_port=0x18 -------------->> mendefinisakan bahwa LCD di hubungkan ke PORT B
#endasm#include <lcd.h> --------------->> library untuk fungsi2 akses LCD
**************
// sub rutin saat terjadi interrupt Timer 1
interrupt [TIM1_OVF] void timer1_ovf_isr(void)
{
TCNT1H=0xC2; ----------------------> nilai didapat dari rumus ......
TCNT1L=0xF7; ----------------------> ....agar Timer 1 bernilai 1 detik
detik++;
lcd_clear();
sprintf(buff,"detik %d",detik); --------------> memasukkan karakter-karakter ke variabel buff
lcd_puts(buff); --------------------------->menampilkan karakter-karakter variabel buff ke LCD
}
Saat kita ingin menampilkan sederet tulisan ke LCD maka kita harus memasukkan karakter-karakter tulisan itu ke suatu variabel array (dalam program di atas adalah variabel "buff"). Baru kemudian data yg ada di variabel array kita tampilkan ke LCD
***************
void main(void)
{// Timer/Counter 1 initialization
// Clock source: System Clock
// Clock value: kHz
// Mode: Normal top=FFFFh
// OC1A output: Discon.
// OC1B output: Discon.
// Noise Canceler: Off
// Input Capture on Falling EdgeTCCR1A=0x00;
TCCR1B=0x04; ------------------> prescaler 256
TCNT1H=0xC2; ------------------> nilai didapat dari rumus ......
TCNT1L=0xF7; ------------------> ....agar Timer 1 bernilai 1 detik
inget rumus: TCNT = (1+0xFFFF) - (waktu *( XTAL / prescaler) )
waktu yg dinginkan adalah 1 detik , XTAL yg saya pakai adl 4 Mhz dan nilai prescaler=256
Jadi,...............
TCNT= (1+65535)-(1detik * (4.000.000/256))
=65536 - (1detik*15625)
=65536-15625
= 49911 (desimal)
= C2F7 (heksadesimal)
Nilai untuk TCNT yang di dapat dari rumus bernilai 16bit (4 angka Heksadesimal), 2 angka yg di depan kita masukkan ke TCNT1H dan 2 angka yg dibelakang kita masukkan ke TCNT1L
****************
// Timer(s)/Counter(s) Interrupt(s) initialization
TIMSK=0x04; ----------------->Timer/Counter1, Overflow Interrupt Enable
code di atas hanya men set "Overflow Interrupt Timer 1". Interrupt baru aktif saat ada perintah: #asm("sei")
****************
// Analog Comparator initialization
// Analog Comparator: Off
// Analog Comparator Input Capture by Timer/Counter 1: Off
// Analog Comparator Output: Off
ACSR=0x80; ------------> me OFF kan analog comparator
SFIOR=0x00;
jika tidak dipakai, sebaiknya analog comparator di OFF. Untuk menghemat pemakaian daya. Hal ini sangat penting jika sumber daya yg digunakan memakai baterai.
*******************
// LCD module initialization
lcd_init(16); ----------------------> inisialisasi LCD 16*2
*******************
// Global enable interrupts
#asm("sei") ----------------------> meng aktifkan Interrupt-interrupt yg sudah di set sebelumnya
Nah... pada saat ini interrupt Timer 1 aktif
*******************
lcd_putsf("wait..."); --------------> menampilkan tulisan wait.. ke LCD
*********************
while (1)
{
};
Program ini yg dijalankan oleh microcontroller... mikro hanya muter disini di dalam while(1){...};
(inget !!! infinite looping di artikel BASIC I/O ).. Jadi mikro sama sekali tidak mengeksekusi perintah.
lha kok bisa????? bingungg.... ~_~ !
Disinilah bedanya pake Interrupt!!.
saat terjadi Interrupt Timer1, alur program mikro akan meloncat ke:
// sub rutin saat terjadi interrupt Timer 1
interrupt [TIM1_OVF] void timer1_ovf_isr(void){
......................................
......yoww..... program yg ada disini yg dijalanin...
......................................
}
setelah program yg ada di sub rutin INTERRUPT dijalankan, maka alur program mikro akan muter2 lagi di infinite looping
******************
jika ada yg kurang dari program di atas mohon kritik dan sarannya
any questions?? post comment on this blog: http:\\avrku.blogspot.com
or send email to: zigan@ymail.com
CodeVisionAVR C Compiler is copyright by Pavel Haiduc, HP InfoTech s.r.l.
AVR is a registered trademark of Atmel Corporation.
Saturday, February 7, 2009
Semenggoh Wildlife Centre

Map of Semenggoh Wildlife Centre
View Larger Map

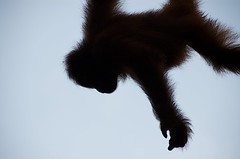
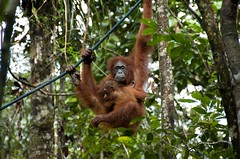
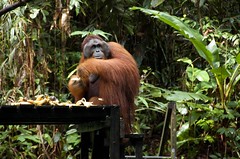

Orang Utans and humans share a long period of post-natal maternal care. Orang Utan mothers take care of their young for a period of around 4 to 5 years after birth, and will only reproduce again when their offspring are independent. Naturally, Orang Utans are semi-solitary animals, and the crowded state of the Semenggoh reserve is only maintained through human assistance. Even with regular feeding times, the 650 hectares of the reserve is only enough room for a single dominant male; currently Ritchie. I wondered how genetic diversity will be maintained in the successfully-breeding captive population, but haven’t yet found out what plans are in place to achieve this. The only mention made of transporting Orang Utans was that Sarawak Forestry supplies them to the zoos in Western Malaysia.
Overall, Semenggoh Wildlife Centre was a great place to visit. We were lucky in seeing so many Orang Utans at the feeding platform. Several guide books mention that sightings are not guaranteed, and that it is worth setting aside a whole day in order to see both morning and afternoon feeding times. The keepers told us that the Orang Utans were more likely to attend feedings when the local plants were not fruiting, so it would be worth checking when this period occurs.
Don’t forget that more pictures of my journey are available online at my Flickr Photostream: http://www.flickr.com/photos/28808691@N05/
Friday, February 6, 2009
Sarawak Cultural Village
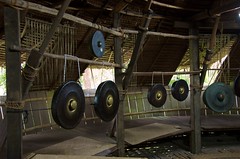
A map of the Sarawak Cultural Village and Damai Beach
View Larger Map
The current population of Sarawak consists mainly of the indigenous Iban people, and immigrant Chinese and Malays. The Iban traditionally lived in groups in the lowlands, in longhouses that were built to last around twenty years. Although they were farmers, the Iban gained notoriety among early European travelers as head-hunters. In some social groups, Iban men were not considered worthy for marriage until they had brought home the head of an enemy from battle. The descendants of the Iban account for around 30% of the current population of Sarawak.




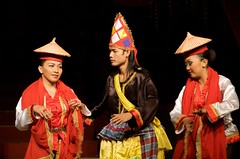
My impression was that the Cultural Village was very much set up to cater to tourists. I think that it represented a good attempt to convey a superficial sense of the culture of the region, but that it was lacking depth in some areas. Aside from the village passport and some posters which were hung in each of the houses, there was little educational material available.
European culture was forced upon Sarawak by James Brooke, an unsuccessful trader, who arrived there in August 1838 after inheriting a sum of £30,000. When he arrived, a Bidayuh uprising was in progress against the Sultan of Brunei, who ruled Sarawak at that time. He helped to induce a peaceful settlement to the uprising and was granted the title of Rajah by the Sultan; thus becoming the first White Rajah.
Don't forget that more pictures of my journey are available online at my Flickr Photostream: http://www.flickr.com/photos/28808691@N05/
Sunday, February 1, 2009
Arrival in Borneo!
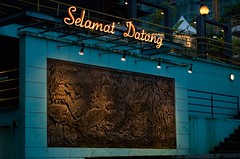
My girlfriend and I arrived in the city of Kuching, on the western coast of the island of Borneo, at 11:30 am yesterday. Borneo itself is split among three nations: Indonesia, Malaysia and Brunei. There are two states in the Malaysian portion; Sabah in the south west and Sarawak (of which Kuching is the capital) in the north west.
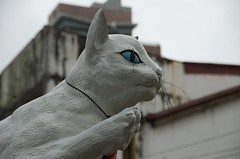
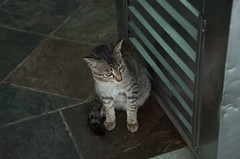
Yesterday afternoon, we were met by another family friend, Uncle Loo, who is a native of Kuching. He took us to visit the weekend market on Jalan Satok (Satok road). Uncle Loo informed us that many of the stall-holders at this market are native river-dwelling people who live further inland than Kuching, and travel to the city only for the weekend market. They sleep on the streets of the market or in their cars. It would be interesting to follow some of these market gardeners back to their homes to see how they live, but that will probably have to wait for a future visit.
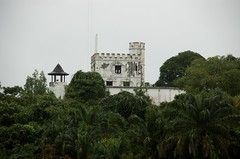
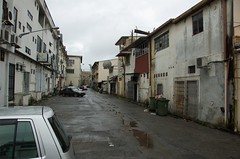
This year, Kuching has suffered unseasonally-long monsoon rains. The areas surrounding the city have been flooded, and local newspapers have reported that around 800 people have been displaced from their homes due to the flooding. It has rained almost continually since we arrived yesterday, but the city of Kuching itself doesn’t appear to have any flooded areas. We have also not seen any sign of the problems that the flooding has caused, but that may be due to our staying in the most affluent part of the city.
As a caucasian here, I draw some attention as a relative rarity. The Kuching population seems to be comprised of three main groups: native people, Malays, and Chinese. Caucasians are called Gwei Los by the Cantonese-speaking Chinese and Mat Salleh by the Malays.
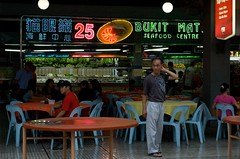
Tomorrow, we are going to the Sarawak Cultural Centre, and hopefully I will have time to write about it in the evening. Don't forget that more pictures of my journey are online at my Flickr photostream: http://www.flickr.com/photos/28808691@N05